π Guides for BasicAI's open and plugin APIs.
OpenAPI
To connect third-party apps with BasicAI for tasks like uploading and downloading annotation data, we provide OpenAPIs. These APIs enable you to create datasets, manage annotation tasks, upload data, and download results. Please review the information below before using the APIs.
Authentication and Security
Authentication
NOTE
When making API calls, remember to include the request header
Authorization: ApiKey xyz
to transmit authentication information, wherexyz
is the API Key created earlier. The API Key is specific to a team and user, and access rights for each API request depend on the user's role within the team.
Here's how to create and manage OpenAPIs in BasicAI:
First, generate API Keys in the Profile Center located at the top right corner of the BasicAI Cloud platform. You can choose the associated Team
and set the Expiration Date
.
Please note that your secret API keys will not be displayed again once you close the settings dialog. Please make sure you have copied them.
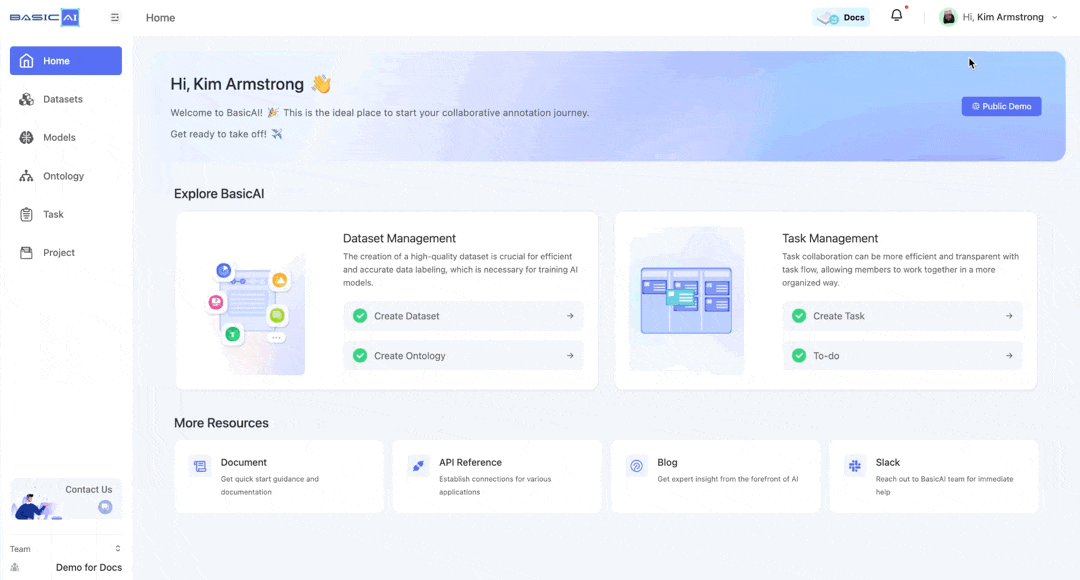
Team administrators can revoke created API Keys in the Team Center from the top right corner at any time.
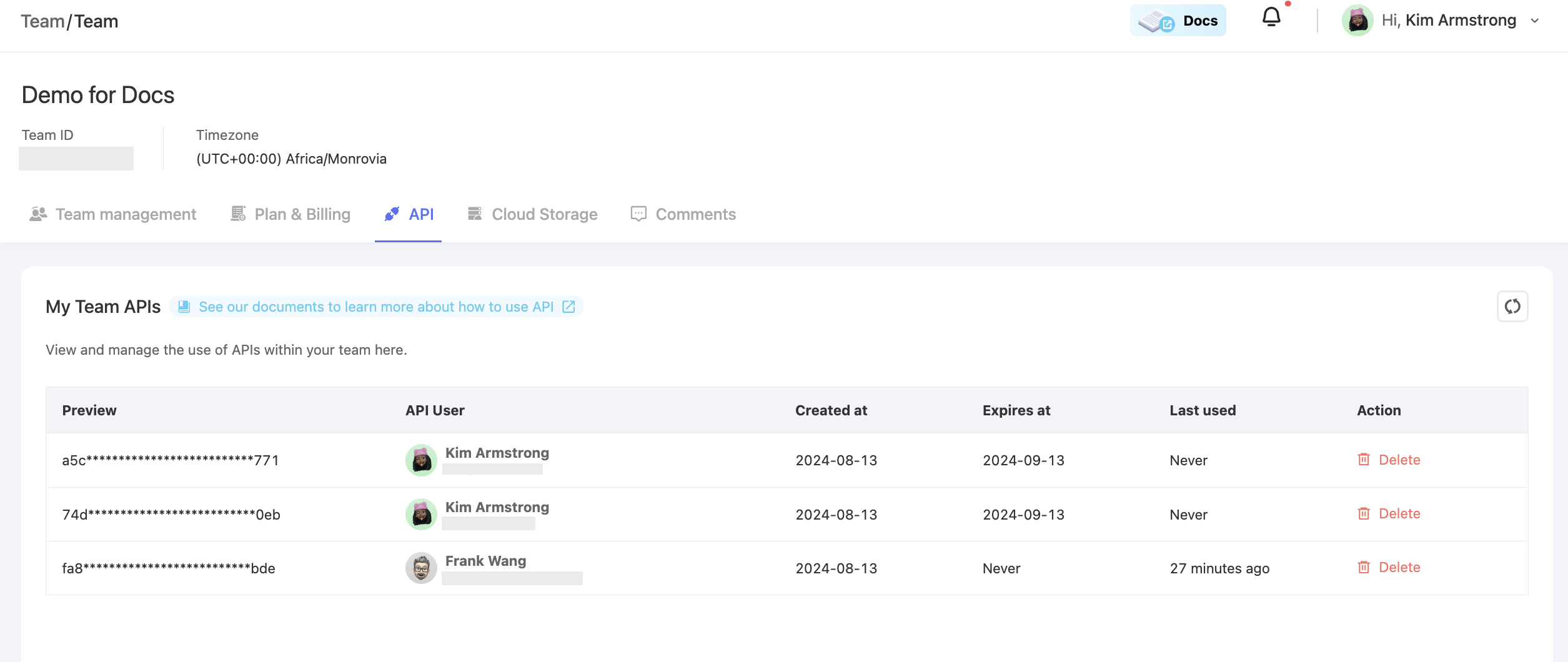
Rate Limiting
To ensure service stability, we have imposed limits on the frequency of API calls for each API Key:
- Not more than 100 times per second
- Not more than 3000 times per minute
- Not more than 72000 times per hour
- Not more than 864000 times per day
If you need to process a large amount of data, please consider using batch operation interfaces to reduce the number of API calls.
File Upload
In annotation scenarios with a large number of files (e.g., images, point clouds, videos), these will be stored in an object storage service such as AWS S3.
For better performance and efficiency, the APIs do not accept file contents directly. Clients need to upload files first and then call the API to submit details such as path, size, and MIME type along with other data.
There are two upload methods, each suited for different use cases.
Pre-signed Upload URLs
Suitable for uploading small files (up to 1GB).
First, call the pre-signed upload address generation API to get an upload address, then use the form-data format to PUT the file content to that address.
Temporary Access Tokens (coming soon)
Suitable for uploading large files (over 1GB) in chunks, this method requires the use of the AWS S3 SDK.
First, call the temporary authorization API to obtain an STS Token for temporary access to the object storage service. Then, use this token with the SDK to upload files, which provides advanced features like chunked uploading to simplify client calls.
OpenAPI Specification
All APIs follow the REST (representational state transfer) style, with necessary adjustments for application scenarios in BasicAI.
Naming Convention
The naming convention for properties of paths, parameters, request bodies, response bodies, and properties of JSON objects should follow the camelCase style with the initial letter in lowercase.
Character Encoding
Request and response bodies should consistently use UTF-8 as their character encoding.
Request Methods
We use only the GET and POST request methods to simplify the user experience, excluding third-party object storage services for file uploads.
The POST method will be used for creating, updating, and deleting operations, with the specific action indicated within the path.
Paths and Parameters
When using REST methods, the path specifies the operation to be performed and includes important resource ID parameters. A maximum of 100 IDs can be passed through Query parameters due to URL length restrictions.
Here is a summary of the defined paths and parameters for the OpenAPI:
- Creating, Updating, and Deleting Datasets:
POST
/dataset/create: Create a dataset.POST
/dataset/update/{datasetId}: Update a dataset with partial data. Only fields provided in the request will be updated.POST
/dataset/replace/{datasetId}: Replace a dataset entirely. Fields not provided in the request will be cleared.POST
/dataset/delete/{datasetId}: Delete a dataset.POST
/dataset/delete?datasetIds=1,2,3: Delete datasets in bulk.
- Querying Datasets:
GET
/dataset/list: Query a list of datasets with support for complex filtering and sorting.GET
/dataset/listById: Query a list of datasets by ID to ensure data continuity across multiple requests, ideal for scenarios requiring traversal and processing of all data.GET
/dataset/info/{datasetId}: Query information for a single dataset.GET
/dataset/info?datasetIds=1,2,3: Query information for datasets in bulk.
- Managing Data within Datasets:
POST
/dataset/addData/{datasetId}: Add data to a dataset.POST
/dataset/removeData/{datasetId}/{dataId}: Remove data from a dataset.POST
/dataset/batchAddData/{datasetId}: Add data in bulk to a dataset.POST
/dataset/batchRemoveData/{datasetId}?dataIds=1,2,3: Remove data in bulk from a dataset.
- Managing Scenes and Batches within Datasets:
POST
/dataset/addScene/{datasetId}: Add a scene under the dataset, supporting the addition of frames underneath simultaneously.POST
/dataset/removeScene/{datasetId}/{sceneId}: Remove a scene under the dataset, cascading to remove frames underneath.POST
/dataset/addBatch/{datasetId}: Add a batch under the dataset, not supporting the addition of nested items simultaneously.POST
/dataset/removeBatch/{datasetId}/{batchId}: Remove a batch under the dataset, only allowing the deletion of empty batches.
- Querying Items within Datasets:
GET
/dataset/listItem/{datasetId}: Query the list of items (data, scenes, or batches) under the dataset, returning only the items at the current level.GET
/dataset/listItemById/{datasetId}: Query the list of items (data, scenes, or batches) under the dataset in ID order to ensure data continuity across multiple requests, ideal for scenarios requiring traversal and processing of all data.
- Querying Annotation Results:
GET
/dataset/listAnnotationSource/{datasetId}: Query the list of annotation sources for the dataset.GET
/dataset/dataAnnotationResult/{datasetId}/{dataId}: Query the annotation results of the data under the dataset. If no source is specified, return all results.GET
/dataset/batchDataAnnotationResult/{datasetId}/?dataIds=1,2,3: Batch query the annotation results of data under the dataset. If no source is specified, return all results.GET
/dataset/sceneAnnotationResult/{datasetId}/{sceneId}: Query the annotation results of scenes under the dataset. If no source is specified, return all results.
API Versioning
The API paths include a version number, for example, /api/v1/dataset/create
, where v1
represents the version. When there is an incompatible upgrade, the version number will be incremented, and the old version will be retained for a period for a smooth transition.
Request Body
The request body is a JSON object.
{
"username": "jack",
"password": "12345678",
"age": 17
}
Response Body
The response body is a JSON object with the following structure:
{
// Business error code
"code": "OK",
// Business error description
"message": "",
// Business data
"data": null
}
Please note that the standard structure is guaranteed only when the response status code is
200
.
Batch Retrieval of List Data
Large sets of data that cannot be retrieved all at once should be returned in batches through multiple requests.
- Pagination:
Suitable for situations where data needs to be retrieved by page.
Note that if the server-side data changes between multiple requests, it may result in data duplication or loss between pages.
Request Parameters:
pageNo
: Page number, default is 1pageSize
: Number of records per page, range 1-100, default is 10
Response Result:
{
"code": "ok",
"message": "",
"data": {
// Total, only return in first page
"total": 100,
// Records
"list": []
}
}
- Traversing in ID Order:
Suitable for scenarios requiring iterative processing of all data, returning records in ID order. This method ensures data continuity between multiple requests and provides better performance.
Request Parameters:
lastId
: ID of the last record returned in the previous request. The response will continue from the record following this ID. If not specified, it starts from the beginning.limit
: Number of records to return, range 1-100, default is 10
Response Result:
{
"code": "ok",
"message": "",
"data": {
// Records
"list": []
}
}
If the number of returned list records (which could be empty) is less than the
limit
specified in the request parameters, it indicates that all records have been retrieved.
Response Codes
- HTTP Status Codes:
HTTP status codes only indicate errors at the HTTP protocol level. More specific errors can be found in the code
and message
fields in the response object. Regardless of the operation's success, a unified 200
status code will be returned as long as the request is sent and processed.
200
OK: Operation successful400
Bad Request: General client error401
Unauthorized: Not authenticated403
Forbidden: Not authorized404
Not Found: Resource not found405
Method Not Allowed: Request method not supported429
Too Many Requests: Too many requests500
Internal Server Error: Internal server error502
Bad Gateway: Error when requesting an upstream service through a gateway503
Service Unavailable: Service unavailable, such as during restart or maintenance504
Gateway Timeout: Timeout when requesting an upstream service through a gateway
- Response Object Codes:
Regardless of the success of the operation, it is important to check whether the error code
in the response JSON object is OK
for success, and any other code for failure.
Common response error codes and messages can be found below:
Code | Description |
---|---|
OK | Success |
ERROR | Error |
RESOURCE_NOT_FOUND | Resource not found |
RESOURCE_DUPLICATED | Resource duplicated |
NAME_DUPLICATED | Name duplicated |
ILLEGAL_PARAM | Illegal parameter |
ILLEGAL_FORMAT | Illegal format |
FILE_FORMAT_NOT_SUPPORTED | File format not supported |
OPERATION_FAILED | Operation failed |
LICENSE_EXPIRED | License expired |
Plugin API (Model Integration)
BasicAI supports custom model integration via plugin API, tailored to meet your unique model annotation requirements.
Head to the Model Center - My Models. You can create a model by yourself or by Hugging Face. For detailed steps, please refer to the Model Guide.